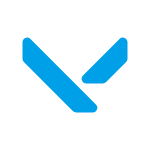
Vertex刷流部分规则
做种6小时上传低于1M.js
(maindata, torrent) => {
const { state, upspeed, completedTime, category } = torrent;
// 排除的分类
const excludeCategories = ["IYUU自动辅种", "keep", "KEEP"];
if (excludeCategories.includes(category)) {
return false;
}
// 只检查已完成的种子状态
const completedStates = ["uploading", "stalledUP"];
if (!completedStates.includes(state)) {
return false;
}
// 检查是否做种超过6小时
const sixHoursInSeconds = 6 * 3600;
if (moment().unix() - completedTime < sixHoursInSeconds) {
return false;
}
// 检查上传速度是否低于1M
if (upspeed >= util.calSize(1, "MiB")) {
return false;
}
return true;
};
站点已删除种子
(maindata, torrent) => {
const { trackerStatus } = torrent;
// 站点删除种子的错误信息
const deletedMessages = [
"torrent banned",
"Torrent not exists",
"torrent not registered with this tracker",
"unregistered torrent",
"Invalid Torrent:"
];
// 如果trackerStatus存在且包含任意一个错误信息,则返回true表示可以删除
if (trackerStatus) {
const trackerMessage = trackerStatus.toLowerCase();
return deletedMessages.some(msg => trackerMessage.includes(msg.toLowerCase()));
}
return false;
};
做种大于20人 下载小于20人 速度低于1M
(maindata, torrent) => {
const categoryList = ["keep", "KEEP"];
const { category, seeder, leecher, uploadSpeed, state } = torrent;
if (categoryList.indexOf(category) !== -1) {
return false;
}
if (state === "downloading") {
return false;
}
if (
seeder > 20 &&
leecher < 20 &&
uploadSpeed < util.calSize(1, "MiB")
) {
return true;
}
return false;
};
辅种3天3倍删除
(maindata, torrent) => {
const { category, uploaded, size, addedTime, tracker } = torrent;
// 只检查IYUU自动辅种分类
if (category !== "IYUU自动辅种") {
return false;
}
// 检查是否是指定的站点
const trackerDomains = ["m-team", "hdsky", "ptchdbits", "hhclub", "chdbits", "rainbowisland"];
const currentTracker = tracker.toLowerCase();
if (!trackerDomains.some(domain => currentTracker.includes(domain))) {
return false;
}
// 检查是否超过3天
const threeDaysInSeconds = 3 * 24 * 3600; // 3天的秒数
if (moment().unix() - addedTime >= threeDaysInSeconds) {
return false;
}
// 检查分享率是否大于3
if (uploaded / size >= 3) {
return true;
}
return false;
};
磁盘小于200G且上传低于500K
(maindata, torrent) => {
const { state, upspeed, completedTime, category } = torrent;
const { freeSpaceOnDisk } = maindata;
// 排除的分类
const excludeCategories = ["IYUU自动辅种", "keep", "KEEP"];
if (excludeCategories.includes(category)) {
return false;
}
// 检查磁盘剩余空间是否小于200G
if (freeSpaceOnDisk >= util.calSize(200, "GiB")) {
return false;
}
// 只检查已完成的种子状态
const completedStates = ["uploading", "stalledUP"];
if (!completedStates.includes(state)) {
return false;
}
// 检查是否做种超过2小时
const twoHoursInSeconds = 7200;
if (moment().unix() - completedTime < twoHoursInSeconds) {
return false;
}
// 检查上传速度是否低于500K
if (upspeed >= util.calSize(500, "KiB")) {
return false;
}
return true;
};
磁盘小于100G且上传低于1M
(maindata, torrent) => {
const { state, upspeed, completedTime, category } = torrent;
const { freeSpaceOnDisk } = maindata;
// 排除的分类
const excludeCategories = ["IYUU自动辅种", "keep", "KEEP"];
if (excludeCategories.includes(category)) {
return false;
}
// 检查磁盘剩余空间是否小于100G
if (freeSpaceOnDisk >= util.calSize(100, "GiB")) {
return false;
}
// 只检查已完成的种子状态
const completedStates = ["uploading", "stalledUP"];
if (!completedStates.includes(state)) {
return false;
}
// 检查是否做种超过2小时
const twoHoursInSeconds = 7200;
if (moment().unix() - completedTime < twoHoursInSeconds) {
return false;
}
// 检查上传速度是否低于1M
if (upspeed >= util.calSize(1, "MiB")) {
return false;
}
return true;
};
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 solitud.es
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果